· Jack Young · Education · 9 min read
Unit 6 (P5, M1, D1): Principles of Software Design
Explain the role of software design principles and software structures in the IT systems development lifecycle. Explain the importance of the quality of code and discuss the factors that can improve the readability of code.
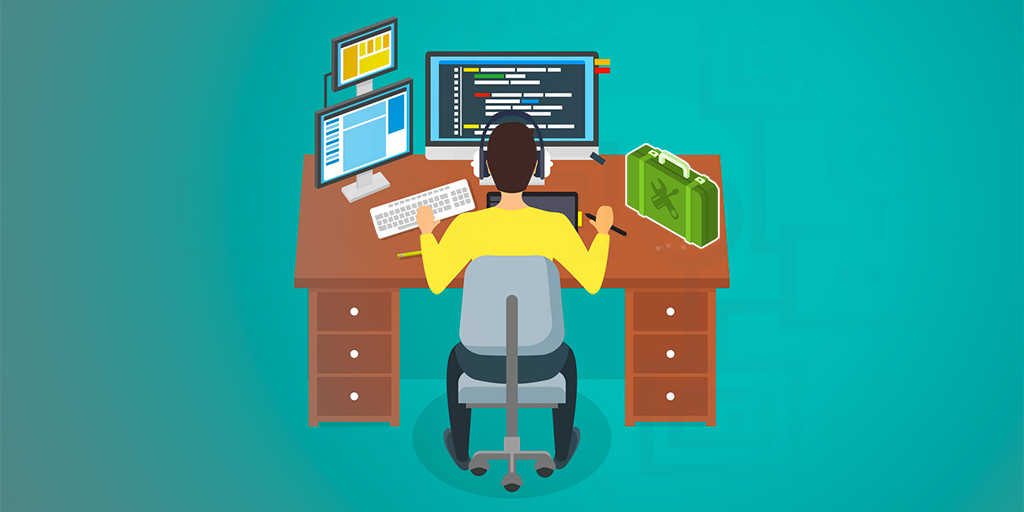
Software Development Lifecycle
Explain the Role of Software Design Principles and Software Structures in the IT Systems Development Lifecycle (P5)
Software Design Principles
Requirements Gathering and Specification
This phase is critical to the success of the project. The expectations of a client or your team need to be written out in detail and documented as well as what are the requirements for the project. There needs to be lots of communication between stakeholders, end users and the project team to make the project successful.
Design
Design requirements are prepared in this phase by lead development staff that can include architects and lead developers. The requirements listed in the previous phase are used to define how the application will be written. Technical requirements will detail database tables to be added, new transactions to be defined, security processes and hardware and system requirements.
Code
This phase is the coding and testing of the process by the development team. After each stage, the developer may demonstrate the work accomplished to the business analysts and tweaks and enhancements may be required. It is important in this phase for developers to be open-minded and flexible if any changes are introduced. This is normally the longest phase. The finished product here is input to the testing phase.
Test
Once the application is migrated to a test environment, different types of testing will be performed including integration and system testing. User acceptance testing is the last part of testing and is performed by the end users to ensure the system meets their expectations. At this point, bugs may be found and more work may be required in the analysis, design or coding. Once sign-off is obtained by all relevant teams, implementation and deployment can begin.
Maintain
The size of the project will determine the complexity of the deployment. Training may be required for end users, operations and on-call IT staff. Roll-out of the system may be performed in stages starting with one branch then slowly adding all locations or it could be a full blown implementation.
One of two methods can be followed. Waterfall is the more traditional model and has a well-structured plan and requirements to be followed. This method works well for large projects that may take many months to develop.
The Agile Methodology is more flexible in the requirements, design and coding process and is very iterative. This process works best for smaller projects and expectations of continuous improvement to the application.
Whether you use one over the other will also depend to a large extent on the corporation and skills of the IT dept.
Software Structures
Functions
Programming languages may have built-in functions that are available to the programmer. Python has many different functions that are built-in.
An example of a Python built-in function is the str( ) function. str(object=b’This is an example string.’, encoding=‘utf-8’, errors=‘strict’). This is telling Python that string b is created and that b has the value of a string ‘This is an example string’. It is in utf encoding and errors are strict. This function is very useful in cases that deal with words. If I wanted to print ‘Incorrect Username or Password’ when someone inputs an invalid value into the login section, then I would be using the str( ) function to do that. Also, the default for errors is ‘strict’, meaning that encoding errors raise a UnicodeError. Other possible values are ‘ignore’, ‘replace’, ‘xmlcharrefreplace’, ‘backslashreplace’ and any other name registered via codecs.register_error().
Procedures
A procedure is a section of a program that performs a specific task.
This is an example of an event driven program in Python, also known as a procedure.
import turtle
turtle.setup(400,500) # Determine the window size
wn = turtle.Screen() # Get a reference to the window
wn.title("Handling key presses!") # Change the window title
wn.bgcolor("lightgreen") # Set the background colour
tess = turtle.Turtle() # Create our favourite turtle
# The next four functions are our "event handlers".
def h1():
tess.forward(30)
def h2():
tess.left(45)
def h3():
tess.right(45)
def h4():
wn.bye() # Close down the turtle window
# These lines "wire up" key presses to the handlers we've defined.
wn.onkey(h1, "Up")
wn.onkey(h2, "Left")
wn.onkey(h3, "Right")
wn.onkey(h4, "q")
# Now we need to tell the window to start listening for events,
# If any of the keys that we're monitoring is pressed, its
# handler will be called.
wn.listen()
wn.mainloop()
This shows that a window will open which has a width of 400 and height of 500. The title of the window which is displayed on the top of it will be called ‘Handling key presses’. The background colour of the window will be light green. The key presses are then defined in the next step; up, left and right keys are to move and the q key is to quit the window.
This is an example of a procedure since when it is triggered; it performs a task of opening a window and listens for certain key presses.
Classes and Objects
Classes are a program building block which provides a foundation for the creation of objects. Object orientation links the data and functions together. Objects are physical things like a computer, keyboard and mouse.
Predefined Code
Many different software systems share similar requirements and it may be that some functions can use procedures or code from previous projects. Using a pre-defined code can save a lot of time as the procedures have already been written and tested.
All of these link into the coding of the software development life cycle. This is where the developers write the actual code that will be implemented into the software. The designing happened before this stage so by now the developers should know what data types they are using and their end goal.
Quality of Code
Explain the Importance of the Quality of Code (M1)
Reliability
In order for code to be reliable, it will need to function every time it is run. If a code breaks down after several tries at running it, then it will not be reliable. Sometimes when you run your code, it may produce errors, this means that something is not working within the code and needs to be fixed in order to make it more reliable. Finally, your code may produce different results even though you are running the same data through it. This means that you may have a code that randomises the data which sometimes may not be needed.
Robustness
To achieve robustness in the code, it will need to handle whatever data is thrown at it. The code needs to have a variety of data types to handle these different data. It also needs to handle errors in the dataset, can it handle blanks in the dataset. So if a data value comes in and its value is 0, will it crash the code or can it handle this value and values like these, like 1.5 or 1. Your code also needs to be able to detect errors and mitigate them to further improve the code.
Usability
To make your code usable, it needs to be easy to use, if you have to keep tweaking your code to make it work then it is not really usable. In addition to this, your code needs to be able be easy to edit by an untrained user. If your code is unstructured and confusing, then this decreases the usability of the code.
Portability
Can your code be used on different computers and/or different operating systems? If it cannot, then depending on what systems you intended it to be on, your code needs to be adjusted to support those systems. In addition to this, can your code be used in different countries? Again, if it cannot, then your code is not portable.
Maintainability
Your code needs to be maintainable. It needs to be easy to edit the code. Putting comments to define what the code means can help other developers know what the code is about and be able to maintain it more efficiently. Also, does the code need to be updated regularly? If so, maybe the code needs to be changed so it lasts longer without having to maintain it often. Separating the UI and the business logic in the code can help make quick changes to the UI without having to redo the code.
This links in with the designing stage of the software development life cycle. This is where the business should design how the code ultimately is. For example, will the code need to be portable? Questions like this needs to be asked when designing the software so the developer has enough information to go on when in the coding stage.
Improving the Readability of Code
Discuss the Factors That Can Improve the Readability of Code (D1)
Comments
Assume whoever is reading your code knows nothing about what it is supposed to do. Your comments should explain every step. All of your algorithms should be well commented since it may be complicated for others to understand. In addition to this, you should try to describe what purpose the code has. For example, instead of writing “add one to variable”, say why this is important in the code, as anyone can see it is adding one to the variable, e.g. “increase the number of page hits by 1”.
Appropriate Names for Variables
Objects should either reference the domain of the problem or standard software artefacts. A List is a List. You may have to create a Customer List which subclasses or implements a List. Variables and function parameters should have descriptive names.
Indentation
This makes it so that it is obvious that a chunk of code is separate from another. This can help when writing a long method. Indenting inside ‘if’ statements and ‘loops’ will make it easy to read. This technique is called nesting, which it shows a chunk of code is “nested” within another chunk of code, and allows the reader to easily follow the nesting pattern.
Improving the code goes into the testing and maintenance stage of the software development life cycle. Developers and IT users who will maintain the code must test first before they make an official release. Sometimes this requires them to improve the code by making it neater and easier to read, or making it more efficient and use less code so it is optimised to run faster.